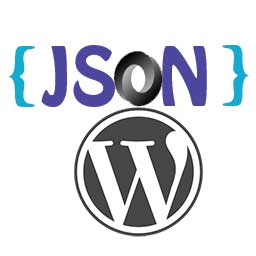
Implementing AJAX in WordPress can provide a lot of flexibility. In addition to the obvious benefits of AJAX form submissions, you can load specific details based on a user’s interaction with the site, making you site more… wait for it… interactive. If nothing else, you can load a lot less data on the initial page load by loading certain pieces asynchronously, helping you to significantly decrease your initial load time.
Implementing AJAX in WordPress through the stock methods is not particularly difficult, but it can be rather tedious. First, you need to create a php function that will return the results you want to load, then you have to hook it into the wp_ajax_no_priv_(action). Then, you have to create a javascript file, enqueue it, and be sure to use wp_localize_script to pass it the location of admin_ajax.php, and be sure to formulate your ajax call to use the appropriate action name. After that, you need to go back to your javascript and be sure to use the ajax response appropriately.
When you need to save something in the database, this is probably the best way to handle things. The admin_ajax.php file has safeguards to ensure that someone else doesn’t hijack it and use it to insert malicious or spammy data in your database. If you only need to display content excerpts, for example, you can leverage the JSON API and do everything in jQuery. The JSON API is a REST web service, so any parameters can be passed entirely through the url structure, and, as the name implies, the results are returned in JSON format, which, as you may have guessed, is very easy to work with in Javascript.
For starters, we need to download and install the JSON API plugin. Now, let’s take a look at the settings – as you can see, there are a few controllers that can be enabled or disabled as needed. By default, the core controller is enabled, which provides read-access for posts, pages, categories, and tags.
So, let’s start with the get_posts method – /api/get_posts/ returns the following:
As you can see, there’s quite a lot of useful data here – beyond the title, content and excerpt, we also have the categories, the tags, and the associated images listed – in every size.
There’s not much here in the way of filtering, and there’s nothing out of the box for listing custom post types or taxonomies; however, you can specify an individual post type by post id or by slug. What’s even better is that it’s completely extensible. You can create custom controllers to do just about anything you want with super complex queries if your heart so desires. This is, perhaps, the best documented plugin in the WordPress plugin repository. The “Other Notes” section alone is 18 pages long.
With get_posts, we can pass count (posts per page), the page number, and the post_type as parameters. With get_post, we can pass the post_id or post_slug and (optionally) the post_type. This makes it ideal for displaying data related to WooCommerce products, for example. Now, let’s try accessing the api via jQuery. In this instance, I’m looking at the Purchase Order Payment Gateway product using:
[code]jQuery.getJSON(‘/api/get_post/?post_type=product&post_slug=woocommerce-purchase-order-payment-gateway’)[/code]
As you can see, there’s quite a bit to work with here – all nice and organized for us. If I want to get the excerpt, I can just use resp.excerpt. For the main thumbnail image, I can use resp.thumbnail. We can traverse the object by expanding the different elements. So, if you wanted to check out the thumbnail image sizes, you’d expand thumbnail_images